Given an m x n
matrix
, return all elements of the matrix
in spiral order.
Example 1:
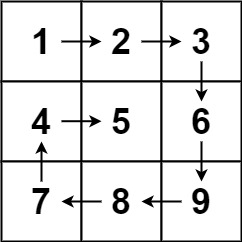
Input: matrix = [[1,2,3],[4,5,6],[7,8,9]] Output: [1,2,3,6,9,8,7,4,5]
Example 2:
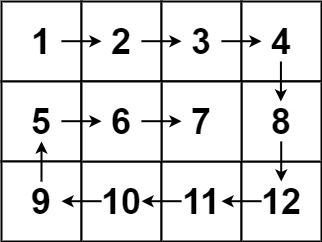
Input: matrix = [[1,2,3,4],[5,6,7,8],[9,10,11,12]] Output: [1,2,3,4,8,12,11,10,9,5,6,7]
Constraints:
m == matrix.length
n == matrix[i].length
1 <= m, n <= 10
-100 <= matrix[i][j] <= 100
Solution :
Given an m x n matrix, return all elements of the matrix in spiral order.
Example 1:
Input: matrix = [[1,2,3],[4,5,6],[7,8,9]]
Output: [1,2,3,6,9,8,7,4,5]
Example 2:
Input: matrix = [[1,2,3,4],[5,6,7,8],[9,10,11,12]]
Output: [1,2,3,4,8,12,11,10,9,5,6,7]
Constraints:
m == matrix.length
n == matrix[i].length
1 <= m, n <= 10
-100 <= matrix[i][j] <= 100
Complete code:
// Cyclically rotate an array by one
#include <bits/stdc++.h>
using namespace std;
/*
IN GCC(C language)
*/
int* spirallyTraverse(int r, int c, int m[][5])
{
int *result = new int[r*c];
static int count = 0;
int i, j=0, k=0;
int r1 = r-1;
int c1 = c-1;
while(count < (r*c))
{
if(count < (r*c))
{
for(i=k; i<=c1; i++)
{
//cout << m[j][i] << " ";
result[count++] = m[j][i];
}
j++;
}
if(count < (r*c))
{
for(i=j;i<=r1; i++)
{
//cout << m[i][c1] << " ";
result[count++] = m[i][c1];
}
c1--;
}
if(count < (r*c))
{
for(i=c1; i>=k; i--)
{
//cout << m[r1][i] << " ";
result[count++] = m[r1][i];
}
r1--;
}
if(count < (r*c))
{
for(i=r1; i>=j; i--)
{
//cout << m[i][k] << " ";
result[count++] = m[i][k];
}
k++;
}
}
return result;
}
/*
For G++ compiler : in C++
*/
vector<int> spiralOrder(vector<vector<int>>& matrix) {
int m = matrix[0].size();
int n = matrix.size();
vector<int> result;
int sr = 0 , er = n-1 , sc = 0 , ec = m-1;
while(sr<=er && sc<=ec)
{
if(sr<=er && sc<=ec)
{
for(int col=sc ; col<=ec ; col++)
result.push_back(matrix[sr][col]);
sr++;
}
if(sr<=er && sc<=ec)
{
for(int row=sr ; row<=er ; row++)
result.push_back(matrix[row][ec]);
ec--;
}
if(sr<=er && sc<=ec)
{
for(int col = ec ; col>=sc ; col--)
result.push_back(matrix[er][col]);
er--;
}
if(sr<=er && sc<=ec)
{
for(int row = er; row>=sr; row--)
result.push_back(matrix[row][sc]);
sc++;
}
}
return result;
}
/*
Another approach
*/
vector<int> spirallyTraverseInCPP(vector<vector<int>>& matrix)
{
vector<int> result;
static int count = 0;
int i, j=0, k=0;
int totalElement = matrix.size() * matrix[0].size();
int r1 = matrix.size()-1;
int c1 = matrix[0].size()-1;
while(count < (totalElement))
{
if(count < (totalElement))
{
for(i=k; i<=c1; i++)
{
result.push_back(matrix[j][i]);
count++;
}
j++;
}
if(count < (totalElement))
{
for(i=j;i<=r1; i++)
{
result.push_back(matrix[i][c1]);
count++;
}
c1--;
}
if(count < (totalElement))
{
for(i=c1; i>=k; i--)
{
result.push_back(matrix[r1][i]);
count++;
}
r1--;
}
if(count < (totalElement))
{
for(i=r1; i>=j; i--)
{
result.push_back(matrix[i][k]);
count++;
}
k++;
}
}
return result;
}
int main()
{
// It is for GCC function(spirallyTraverse)
/*int matrix[][5] = {{6,6,2,28,2},
{12,26,3,28,7},
{22,25,3,4,23}};
int r = 3, c = 5;
int *res = spirallyTraverse(r, c, matrix);
for(int i = 0; i<(r*c); i++)
cout << res[i] << " ";
cout << endl;
delete[] res;*/
vector<vector<int>> vect = {{1,2,3,4},
{5,6,7,8},
{9,10,11,12}};
int totalElement = vect.size() * vect[0].size();
//vector<int> res = spirallyTraverseInCPP(vect);
vector<int> res = spiralOrder(vect);
for(int i = 0; i<totalElement; i++)
cout << res[i] << " ";
return 0;
}