A loop statement allows programmers to execute a statement or group of statements multiple times without repetition of code. In other hand, Loops are used to repeat a block of code until the specified condition is met.
Why we need loops?
If we need to print the table of 2, we can use multiple print statement.// C program to illustrate need of loops
#include <stdio.h>
int main()
{
printf( "2\n");
printf( "4\n");
printf( "6\n");
printf( "8\n");
printf( "10\n");
printf( "12\n");
printf( "14\n");
printf( "16\n");
printf( "18\n");
printf( "20\n"); return 0;
}
In other hand, we can use loop and print the table. There will be less line of code.// C program to illustrate need of loops
#include <stdio.h>
int main()
{
for(int i=1; i<=10; i++)
{
printf( "i*2\n");
}
return 0;
}
There are mainly two types of loops:
- Entry Controlled loops: In Entry controlled loops the test condition is checked before entering the main body of the loop. For Loop and While Loop is Entry-controlled loops.
- Exit Controlled loops: In Exit controlled loops the test condition is evaluated at the end of the loop body. The loop body will execute at least once, irrespective of whether the condition is true or false. do-while Loop is Exit Controlled loop.
Types of Loop in C
There are 3 types of Loop in C language, namely:
for
loop : first Initializes, then condition check, then executes the body and at last, the update is done.while
loop : first Initializes, then condition checks, and then executes the body, and updating can be inside the body.do while
loop : do-while first executes the body and then the condition check is done.
1. for loop
The for
loop in C is used to execute a set of statements repeatedly until a particular condition is satisfied. We can say it is an open ended loop.
Syntax :for(initialization; condition; increment/decrement)
{
statement-block;
}
The for
loop is executed as follows:
- It first evaluates the initialization code.
- Then it checks the condition expression.
- If it is true, it executes the for-loop body.
- Then it evaluate the increment/decrement condition and again follows from step 2.
- When the condition expression becomes false, it exits the loop.
Flowchart:
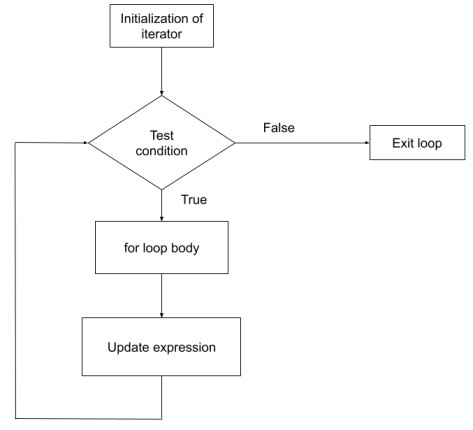
We first initialize our iterator. Then we check the condition of the loop. If it is false, we exit the loop and if it is true, we enter the loop. After entering the loop, we execute the statements inside the for
loop, update the iterator and then again check the condition. We do the same thing unless the test condition returns false.
Example :
Program to print first 10 natural numbers using for
loop
#include<stdio.h>
void main( )
{
int x;
for(x = 1; x <= 10; x++)
{
printf("%d\t", x);
}
}
/*
Output:
1 2 3 4 5 6 7 8 9 10
*/
Nested for
loop :
We can also have nested for
loops, i.e one for
loop inside another for
loop in C language. This type of loop is generally used while working with multi-dimensional arrays.
Syntax:
for(initialization; condition; increment/decrement)
{
for(initialization; condition; increment/decrement)
{
statement ;
}
}
Example:
Program to print half Pyramid of numbers using Nested loops
#include<stdio.h>
void main( )
{
int i, j;
/* first for loop */
for(i = 1; i < 5; i++)
{
printf("\n");
/* second for loop inside the first */
for(j = i; j > 0; j--)
{
printf("%d", j);
}
}
}
/*
Output :
1
21
321
4321
54321
*/
2. While Loop
While loop does not depend upon the number of iterations. If the condition will become false then it will break from the while loop else body will be executed.
The while
loop is an entry controlled loop. It is completed in 3 steps.
- Variable initialization.(e.g
int x = 0;
) - condition(e.g
while(x <= 10)
) - Variable increment or decrement (
x++
orx--
orx = x + 2
)
Syntax:variable initialization;
while(condition)
{
statements;
variable increment or decrement;
}
Flowchart for while loop:
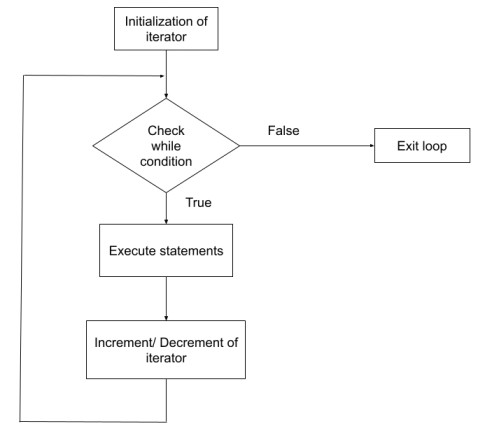
We can see that firstly, we initialize our iterator. Then we check the condition of while
loop. If it is false, we exit the loop and if it is true, we enter the loop. After entering the loop, we execute the statements inside the while
loop, update the iterator and then again check the condition. We do the same thing unless the condition is false.
Program to print first 10 natural numbers using while
loop
#include<stdio.h>
void main( )
{
int x;
x = 1;
while(x <= 10)
{
printf("%d\t", x);
/* below statement means, do x = x+1, increment x by 1 */
x++;
}
}
/*
Output:
1 2 3 4 5 6 7 8 9 10
*/
3. do-while Loop
There is some situation where we execute body of the loop once before testing the condition. Such situations can be handled with the help of do-while
loop.
The do-while loop is similar to a while loop but the only difference lies in the do-while loop test condition which is tested at the end of the body. In the do-while loop, the loop body will execute at least once irrespective of the test condition.
Syntax:
initialization_expression;
do
{
// body of do-while loop
update_expression;
} while (test_expression);
Flowchart for do-while loop:
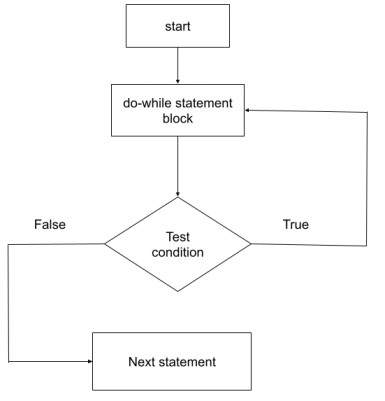
We initialize our iterator. Then we enter body of the do-while loop. We execute the statement and then reach the end. At the end, we check the condition of the loop. If it is false, we exit the loop and if it is true, we enter the loop. We keep repeating the same thing unless the condition turns false.
Example :
Program to print first 10 natural numbers using while
loop
#include<stdio.h>
void main( )
{
int x;
x = 1;
do
{
printf("%d\t", x);
/* below statement means, do x = x+1, increment x by 1 */
x++;
}
while(x <= 10);
}
/*
Output:
1 2 3 4 5 6 7 8 9 10
*/
Infinite Loops
We come across infinite loops in our code when the compiler does not know where to stop. It does not have an exit. This means that either there is no condition to be checked or the condition is incorrect.
// for loop
#include <stdio.h>
int main()
{
for(int i = 0; ; i++)
printf("Infinite loop\n");
return 0;
}
// while loop
#include <stdio.h>
int main()
{
int i = 0;
while(i == 0)
printf("Infinite loop\n");
return 0;
}
// do-while loop
#include <stdio.h>
int main()
{
do{
printf("Infinite loop\n");
} while(1);
return 0;
}
Jumping Out of Loops
while executing a loop, it becomes necessary to skip a part of the loop or to leave the loop as soon as certain condition becomes true.
For jumping out fro the loop, we use break and continue statement.
To learn about break and continue Please check break and continue statement.
7. Jump Statements
7.1 Break
7.2 Continue